Redux store ๐๏ธ
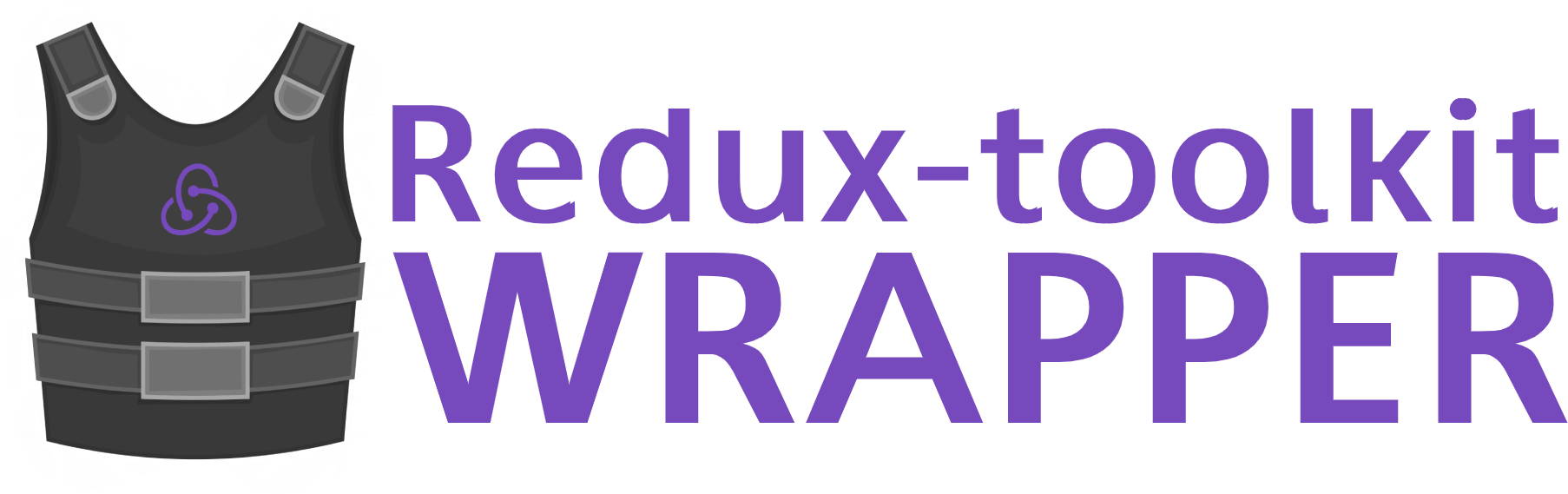
The store section is now really easy to use thanks to Redux-tookit and our Redux-tookit-wrapper.
Architecture
The root file include configuration of redux. The two main constants are reducers
and persistConfig
whitelist
includes state to persist (withredux-persist
)reducers
includes allreducer modules
Slices
A slice is a group of actions, states and reducers for a same domain. For example, in this boilerplate, there are tree slices : Startup
Theme
and User
.
In each slice, an index.js
file which combines each store's feature/module (fetchOne.js
for the User
slice example).
We've decided to separate each module in one file in order to avoid very large incomprehensible files.
So each of them includes its scoped state, his only action and related reducers.
In the index.js
file, all modules are merged in a slice where states, actions, and reducers are merged and placed into it.
For the User
example, the below state will be created :
Actions will be : user/fetchOne/pending
, user/fetchOne/fulfilled
, user/fetchOne/rejected
prefixed and wrapped by the user/fetchOne
action
For each wrapped action, a reducer is associated.
Redux-toolkit-wrapper
The boilerplate includes a wrapper of redux-toolkit to make it easier to use. It provides three helpers. If your are not familiar with redux-toolkit, please have a look at their documentation.
buildAsyncState
buildAsyncState
create a loading and error state. You can scope it in a key.
Parameters | Description | Type | Default |
---|---|---|---|
scope | name of the scope | string | undefined |
Example
Will generate:
buildAsyncActions
buildAsyncActions
is a wrapper of createAsyncThunk
.
Parameters | Description | Type | Default |
---|---|---|---|
actionName | the name of the action | string | undefined |
action | function to launch and await | function | () => {} |
Example
Where fetchOneUserService is an async function.
So, when the fetchOneUserService is launched the action user/fetchOne/pending
is dispatched.
When the fetchOneUserService is endded the action user/fetchOne/fulfilled
is dispatched.
When the fetchOneUserService throw an error the action user/fetchOne/rejected
is dispatched.
buildAsyncReducers
buildAsyncReducers
create default reducers based on CRUD logic. It creates three functions : pending, fulfilled and rejected.
pending
set theloadingKey
totrue
and theerrorKey
tonull
.fulfilled
replacesitemKey
with the payload (ifitemKey
is notnull
) and theloadingKey
tofalse
rejected
set theloadingKey
tofalse
and theerrorKey
to payload.
Parameters | Description | Type | Default |
---|---|---|---|
itemKey | the key of the item state | string | 'item' |
loadingKey | the key of the loading state | string | 'loading' |
errorKey | the key of the error state | string | 'error' |
Example
buildSlice
buildSlice
is a wrapper of createSlice
.
Parameters | Description | Type | Default |
---|---|---|---|
name | the name of the slice | string | undefined |
modules | array of all modules | array | [] |
sliceInitialState | initial state for all modules of the slice | object | {} |
Example
note
For async function you have to use buildAsyncState
, buildAsyncActions
and buildAsyncReducers
from @thecodingmachine/redux-toolkit-wrapper.
For no async function you have to use createAction
from redux-toolkit. And follow the example of the slice Theme